Mastering Data Science Algorithms with Python A Comprehensive Overview
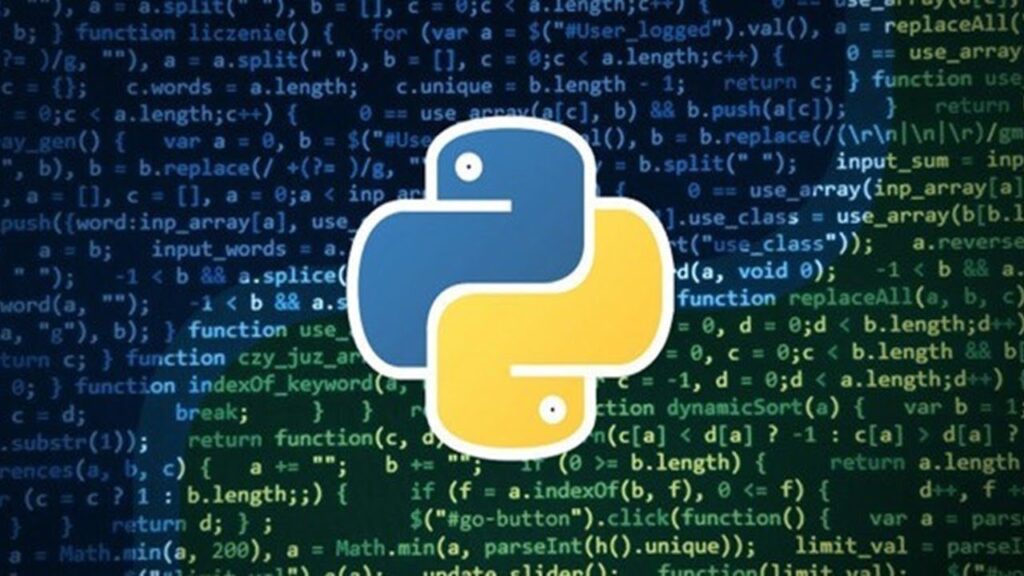
In the realm of data science, algorithms serve as the backbone for extracting insights, making predictions, and uncovering patterns from raw data. Python, with its extensive library ecosystem, provides a robust platform for implementing and experimenting with various algorithms. In this comprehensive overview, we'll delve into a wide array of data science algorithms, covering essential concepts, implementation examples, and practical applications.
Introduction to Data Science Algorithms
Data science algorithms encompass a broad range of techniques used to analyze, interpret, and derive meaning from data. These algorithms can be categorized into several main types, including:
- Supervised Learning Algorithms: Algorithms that learn from labeled data to make predictions or decisions.
- Unsupervised Learning Algorithms: Algorithms that identify patterns and structures in unlabeled data.
- Semi-supervised Learning Algorithms: Algorithms that leverage both labeled and unlabeled data for learning.
- Reinforcement Learning Algorithms: Algorithms that learn by interacting with an environment and receiving feedback.
- Dimensionality Reduction Algorithms: Algorithms that reduce the number of features in a dataset while preserving important information.
Implementing Algorithms in Python
Python's rich ecosystem of libraries, including NumPy, SciPy, Scikit-learn, and TensorFlow, provides a comprehensive toolkit for implementing data science algorithms. Let's explore some examples:
Example 1: Linear Regression (Supervised Learning)
from sklearn.linear_model import LinearRegression
import numpy as np
# Generate synthetic data
X = np.array([[1], [2], [3]])
y = np.array([2, 3, 4])
# Initialize and train the model
model = LinearRegression()
model.fit(X, y)
# Make predictions
predictions = model.predict([[4]])
print(predictions) # Output: [5.]
Example 2: K-Means Clustering (Unsupervised Learning)
from sklearn.cluster import KMeans
import numpy as np
# Generate synthetic data
X = np.array([[1, 2], [1, 4], [1, 0], [4, 2], [4, 4], [4, 0]])
# Initialize and fit the model
model = KMeans(n_clusters=2)
model.fit(X)
# Get cluster labels
labels = model.labels_
print(labels) # Output: [1 1 1 0 0 0]
Example 3: Principal Component Analysis (Dimensionality Reduction)
from sklearn.decomposition import PCA
import numpy as np
# Generate synthetic data
X = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Initialize and fit the PCA model
pca = PCA(n_components=2)
pca.fit(X)
# Transform the data
transformed_data = pca.transform(X)
print(transformed_data)
ractical Applications of Data Science Algorithms
Data science algorithms find applications across various domains, including finance, healthcare, e-commerce, and more. Some common use cases include:
- Predictive analytics for forecasting sales or stock prices.
- Recommender systems for personalized product recommendations.
- Fraud detection in financial transactions.
- Medical diagnosis and disease prediction using patient data.
- Natural language processing for sentiment analysis and text classification.
Deja una respuesta